A beginner’s guide to WordPress hooks
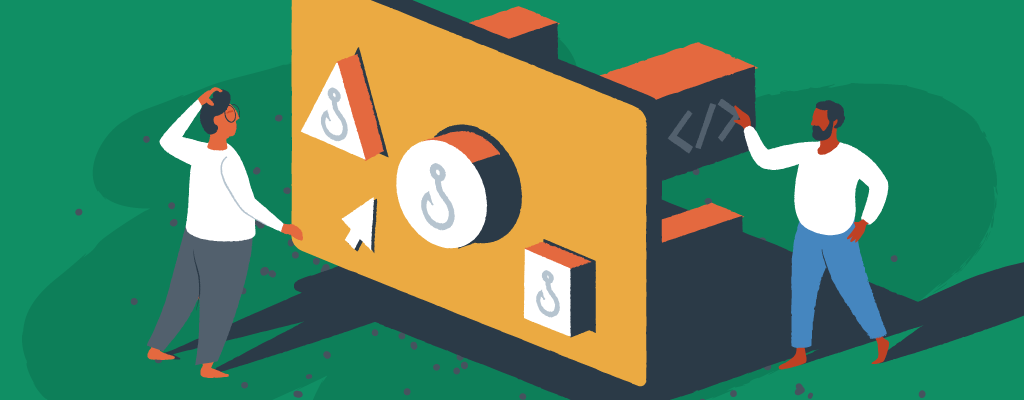
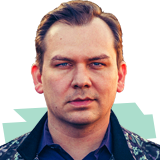
As you get more familiar with WordPress, you’ll start to catch onto its expansive assortment of tools and capabilities. One of these toolsets is an amazing feature called hooks. Any developer looking to customize a website in new ways will benefit from understanding how hooks function and the possibilities available.
This beginner’s guide will walk you through the world of WordPress hooks, explaining what they are, how they work, and how you can use them to enhance any WordPress projects.
Understanding WordPress hooks
WordPress hooks are a hidden secret of the platform’s unrivaled flexibility, letting developers modify designs and functionality without altering the original code. They act as designated points in WordPress where you can insert custom code to add new website features, modify existing ones, or change how WordPress behaves in certain instances.
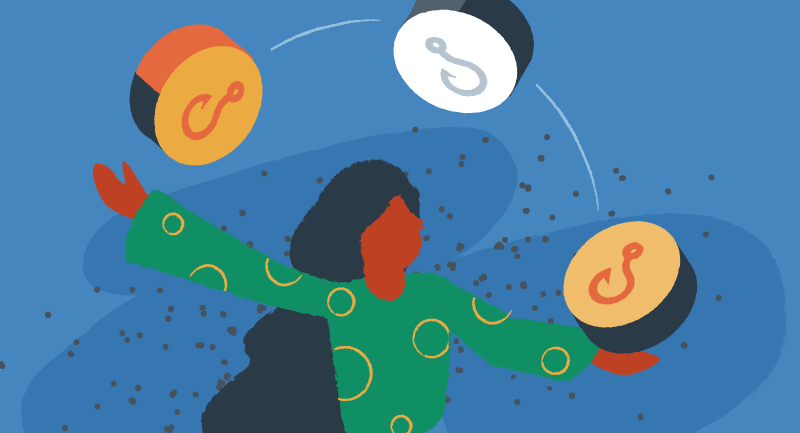
To grasp the concept of hooks, it’s helpful to think of them as “listeners.” WordPress hooks listen for certain points in the code execution and allow developers to run custom functions at those exact moments.
By using hooks, developers can add, remove, or modify your site’s performance without touching the core WordPress files. This approach offers some big advantages:
- Maintainability – When WordPress updates are released, your custom code remains intact since it’s separate from the core files.
- Compatibility – Hooks provide a standardized way for different plugins and themes to interact, reducing conflicts and improving stability.
- Scalability – As your project grows, hooks allow you to add new features or modify existing ones easily.
- Community collaboration – The hook system helps developers create plugins and themes that others can easily extend.
WordPress hooks come in two different types: actions and filters. While both serve the purpose of extending functionality, they operate in slightly different ways:
- Action hooks – These allow you to add or remove processes at specific points. They’re ideal for triggering custom functions when certain events occur, such as when a post is published or a user logs in.
- Filter hooks – These let you modify data as it flows through WordPress. Filters intercept data at certain points, manipulate it, and then return the modified data back to WordPress for further use.
Exploring action hooks
To better understand WordPress action hooks, let’s break down their key characteristics and explore how they function within the WordPress ecosystem:
- Timing-based execution – Action hooks are triggered at predetermined points in the WordPress execution cycle. These points can be during page load when specific events occur (like publishing a post) or at various stages of plugin or theme initialization.
- No return value – Unlike filter hooks, action hooks don’t return a value. Their primary purpose is to perform an action or series of actions when triggered.
- Multiple callback functions – You can attach multiple functions to a single action hook. WordPress will execute these functions in the order they were added.
Here’s a basic example of how an action hook works:
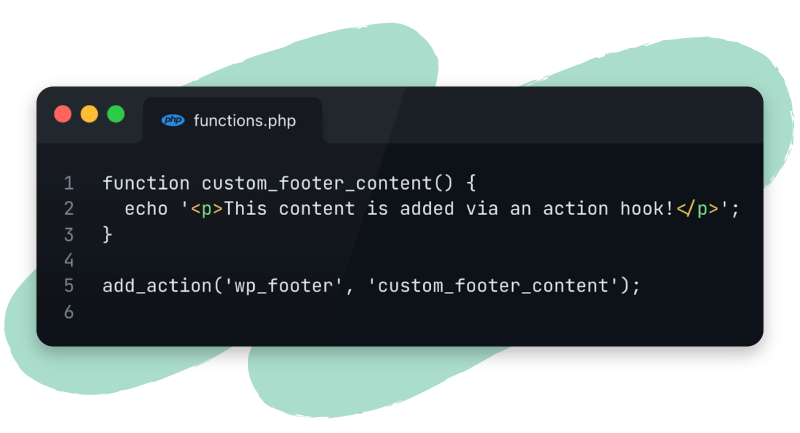
function custom_footer_content() { echo '<p>This content is added via an action hook!</p>';}add_action('wp_footer', 'custom_footer_content');
In this example, we’ve created a function called custom_footer_content() and hooked it to the wp_footer action. This means that whenever WordPress reaches the point where it processes the footer, our custom function will be executed, adding the specified content to the page.
Action hooks are incredibly versatile and can be used for various purposes:
- Content manipulation – Add, remove, or modify content on different parts of your website.
- User interaction – Trigger custom functions when users perform specific actions, like logging in or commenting.
- Plugin and theme integration – Allow your plugins or themes to interact with other WordPress components or third-party extensions.
The WordPress Codex provides a comprehensive list of available hooks, along with documentation on when they’re triggered and what parameters they pass to callback functions.
Diving into filter hooks
WordPress filters are the second major type of hooks, complementing action hooks by providing a way to modify data as it flows through the system. While action hooks allow you to add or trigger new functions, filter hooks help you intercept, manipulate, and return adjusted information.
Let’s explore the key characteristics and functionalities of filter hooks:
- Data modification – The primary purpose of filter hooks is to alter data. They receive a value (or set of values), allow you to modify it, and then return the altered data back to WordPress.
- Return value required – Unlike action hooks, filter hooks must always return a value. This returned value is what WordPress will use for further processing or display.
- Chaining capability – Multiple functions can be attached to a single filter hook. WordPress will pass the data through each function in sequence, with each function potentially modifying the data before passing it to the next.
Here’s a basic example of how a filter hook works:
function custom_excerpt_length($length) { return 30; // Change excerpt length to 30 words}add_filter('excerpt_length', 'custom_excerpt_length');
In this example, we’re using the excerpt_length filter to modify the default excerpt length. Our function custom_excerpt_length() receives the current excerpt length as a parameter, modifies it to 30 words, and returns the new value.
Filter hooks are extensively used in WordPress for various purposes:
- Content formatting – Modify how content is displayed, such as changing the excerpt length or adding custom markup to post content.
- Form input processing – Sanitize or validate user input before it’s saved to the database.
- URL and link modification – Change how URLs are generated or modify link attributes.
- Text translation – Intercept and modify text strings for localization purposes.
The WordPress Codex and developer documentation again provide detailed information about available filter hooks, including what data they pass and what type of value should be returned.
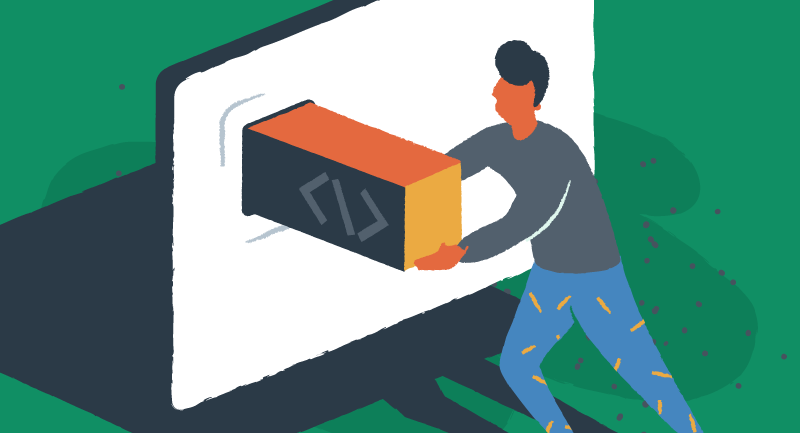
Understanding the difference between action and filter hooks
While both action and filter hooks are essential components of the WordPress hook system, they serve different purposes and are used in distinct scenarios. Understanding the key differences between these two types of hooks is crucial for understanding these tools.
Here’s a comparison of the primary differences:
Aspect | Action Hooks | Filter Hooks |
Purpose | Execute custom code | Modify data |
Return Value | None | Required |
Data Handling | Reference only | Modify and return |
Execution | Independent | Chained |
Typical Use Cases | Adding features, triggering events | Modifying content, altering queries |
Implementing hooks in your WordPress projects
Now that we’ve explored the differences between action and filter hooks let’s dive into how you can implement them in your website projects. Whether you’re building a plugin, developing a theme, or customizing an existing site, understanding how to use WordPress hooks properly is essential for creating flexible and maintainable code.
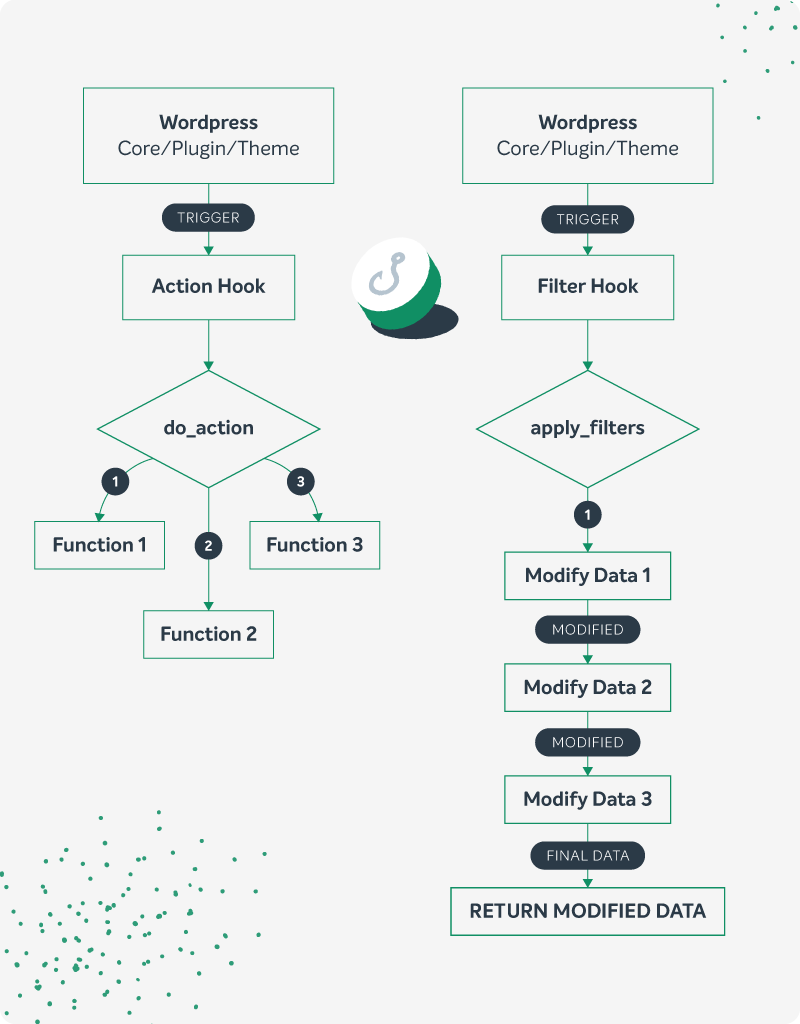
Setting up your development environment
Before you start implementing hooks, ensure you have a proper development environment set up. This typically includes:
- A WordPress installation for testing
- A code editor or IDE with PHP syntax highlighting
- Basic knowledge of PHP and WordPress core functions
Implementing action hooks
To implement an action hook, you’ll use the add_action() function. Here’s the basic syntax:
add_action( 'hook_name', 'callback_function', priority, accepted_args );
Let’s break down each parameter:
- hook_name – The name of the action hook you want to attach your function to.
- callback_function: The name of your custom function that will be executed when the hook is triggered.
- priority (optional) – Determines the order in which your function is executed relative to other functions attached to the same hook. Default is 10.
- accepted_args (optional) – Specifies the number of arguments your callback function accepts. Default is 1.
Here’s an example of using an action hook to add custom content to the footer of your WordPress site:
function add_custom_footer_content() { echo '<div class="custom-footer">'; echo '<p>Thanks for visiting! Follow us on social media:</p>'; echo '<a href="#">Facebook</a> | <a href="#">Twitter</a> | <a href="#">Instagram</a>'; echo '</div>';}add_action( 'wp_footer', 'add_custom_footer_content' );
In this example, we’ve created a function that outputs custom HTML content and hooked it to the wp_footer action. This content will now appear in the footer of every page on your WordPress site.
Implementing filter hooks
To implement a filter hook, you’ll use the add_filter() function. The syntax is similar to add_action():
add_filter( 'hook_name', 'callback_function', priority, accepted_args );
The parameters work the same way as with add_action(), but remember that filter hooks must always return a value.
Here’s an example of using a filter hook to modify the default excerpt length:
function custom_excerpt_length( $length ) { return 25; // Change excerpt length to 25 words}add_filter( 'excerpt_length', 'custom_excerpt_length' );
In this case, we’re intercepting the excerpt_length filter, modifying the length value, and returning the new value to be used by WordPress.
Best practices for implementing hooks
- Ensure your callback function names are unique to avoid conflicts with other plugins or themes.
- Before adding a hook, check if the function already exists to prevent errors:
if ( ! function_exists( 'my_custom_function' ) ) { function my_custom_function() { // Your code here } } add_action( 'init', 'my_custom_function' );
- Use priorities to control the order of execution when multiple functions are attached to the same hook.
- For larger projects, consider using PHP namespaces or classes to organize your hook implementations and avoid naming conflicts.
Essential tools you can rely on
As you delve deeper into WordPress development, you’ll discover that hooks are an essential tool in your toolkit. They provide a standardized way to interact with WordPress core, themes, and plugins, opening up endless possibilities for customization and innovation.
Ready to expand your development know-how even further? Check out our guide to WordPress theme development.