WordPress shortcodes: A guide to creation and implementation
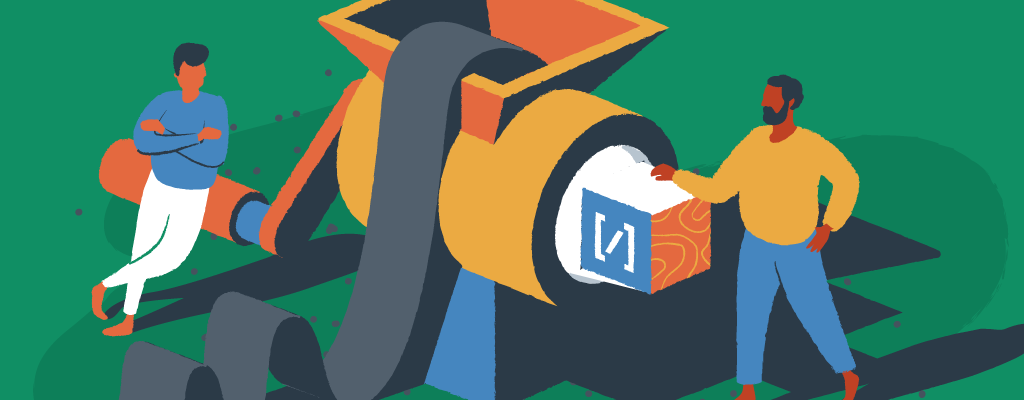
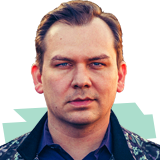
Have you ever wished for a simpler way to add complex functionality to your WordPress website without a lot of bulky code? Enter the world of WordPress shortcodes – your ticket to enhancing your site’s capabilities with minimal effort. In this guide, we’ll explore the ins and outs of these powerful tools, from their basic concepts to advanced implementation techniques.
Whether you’re a curious beginner or a seasoned developer looking to expand your toolkit, we’ll equip you with the knowledge to leverage shortcodes effectively in your WordPress projects.
Understanding WordPress shortcodes
WordPress shortcodes add powerful functionality with just a few keystrokes. At their core, shortcodes are small pieces of code enclosed in square brackets that act as placeholders for more complex functions or content. When WordPress encounters a shortcode in your post or page, it replaces it with the output of the associated function, allowing you to embed dynamic elements without writing elaborate HTML or PHP.
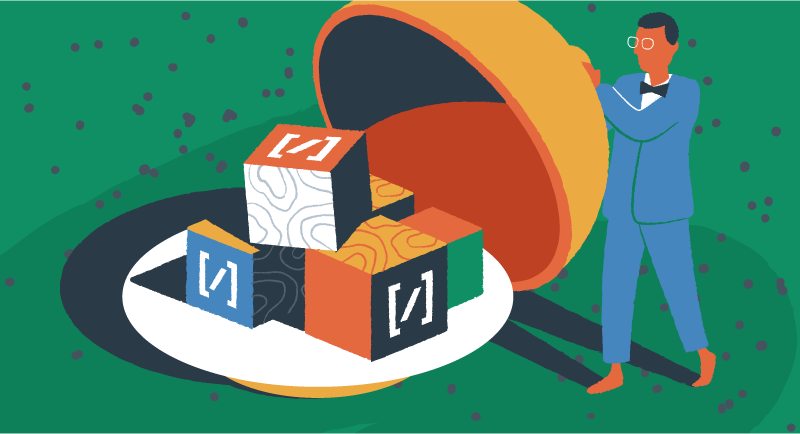
Shortcodes bridge the gap between the user-friendly interface of the WordPress editor and the complex backend functionality that powers your site. With shortcodes, you can easily insert galleries, videos, custom layouts, and even integrate with third-party services – all without leaving the comfort of your content editor.
Let’s break down the anatomy of a typical shortcode:
- Opening bracket – The shortcode begins with a square bracket [.
- Name – This is the identifier for the shortcode, telling WordPress which function to execute.
- Attributes – Optional parameters that modify the shortcode’s behavior, usually in key=”value” format.
- Content – Some shortcodes can wrap around content, affecting how it’s displayed.
- Closing bracket – The shortcode ends with a square bracket ].
Here’s a simple example of a shortcode in action:
[gallery id="123" size="medium"]
In this case, gallery is the shortcode name, while id and size are attributes that customize its output.
The evolution of WordPress shortcodes
Introduced in WordPress 2.5, shortcodes were designed to solve a common problem: how to allow users to easily embed complex functionality within their content without compromising security or requiring extensive coding knowledge.
Before shortcodes, adding dynamic elements to posts often involved directly inserting PHP code into the content. This approach was not only cumbersome but also posed significant security risks. The introduction of shortcodes provided a safer, more accessible alternative that maintained a clear separation between content and functionality.
Benefits of using WordPress shortcodes
Incorporating shortcodes into your WordPress toolkit offers many advantages that can significantly enhance your website’s functionality and content creation process.
- Simplicity and ease of use – Shortcodes allow you to add complex features to your content with just a few characters, making it accessible even for non-technical users.
- Flexibility and customization – Many shortcodes accept parameters, allowing you to tailor their output to suit specific needs without altering the underlying code.
- Reusability – Once created, shortcodes can be used across multiple posts and pages, saving time and effort in content creation.
- Better performance – Shortcodes can help improve page load times by loading only the necessary resources for specific actions rather than loading everything on every page.
- Compatibility with page builders – Many popular page builders support shortcodes, allowing you to integrate custom functionality seamlessly into your designs.
By leveraging these benefits, you can create a more dynamic and engaging website while streamlining your content creation process. As we delve deeper into the world of shortcodes, you’ll discover even more ways to harness their power to enhance your WordPress experience.
Built-in WordPress shortcodes
WordPress has built-in shortcodes that provide essential functionality right out of the box. These pre-defined shortcodes allow you to easily embed common elements into your posts and pages without needing custom code or plugins.
Gallery shortcode
The gallery shortcode allows you to create image galleries effortlessly. You can customize the gallery’s appearance with various attributes:
[gallery ids="123,124,125" columns="3" size="medium"]
This shortcode creates a gallery with specific images, three columns, and medium-sized thumbnails.
Caption shortcode
Use the shortcode to add captions to your images:
[caption id="attachment_123" align="aligncenter" width="300"]Your image description here[/caption]
This wraps your image in a caption container with specified alignment and width.
Audio shortcode
Embed audio files easily with the shortcode:
[audio src="path/to/your/audio-file.mp3"]
You can also specify multiple audio sources for better browser compatibility.
Video shortcode
Similar to audio, you can embed videos using the shortcode:
[video width="640" height="360" src="path/to/your/video-file.mp4"]
This shortcode supports various video formats and allows you to set dimensions.
Playlist shortcode
Create a collection of audio or video files with the shortcode:
[playlist ids="123,124,125" type="audio"]
This creates an interactive playlist of the specified media files.
Embed shortcode
While not always necessary due to WordPress’s auto-embed feature, you can use the shortcode for more control:
[embed width="500" height="300"]https://www.youtube.com/watch?v=dQw4w9WgXcQ[/embed]
This embeds external content like YouTube videos with specified dimensions.
Remember, while these shortcodes are powerful on their own, they’re just the tip of the iceberg. The true potential of shortcodes lies in their extensibility.
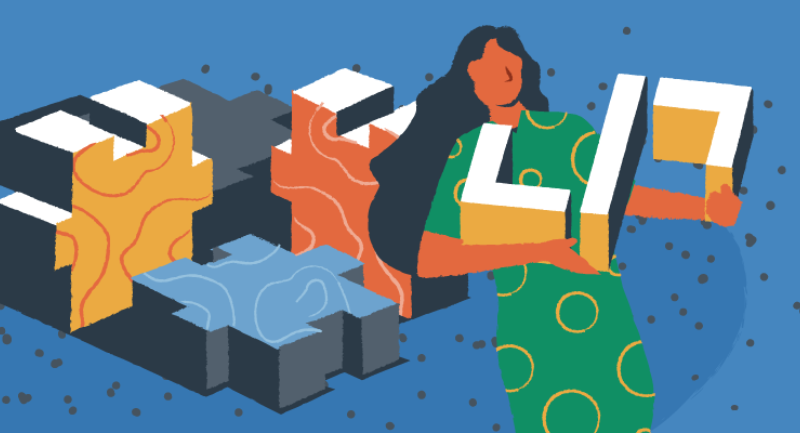
Creating custom WordPress shortcodes
While built-in shortcodes offer great functionality, the real power of WordPress shortcodes lies in the ability to create custom ones tailored to your specific needs. Creating a custom shortcode allows you to encapsulate several functions into a simple, reusable snippet that can be easily inserted into your content.
Define the shortcode function
Start by creating a PHP function that will generate the output for your shortcode. This function should return the content you want to display:
function my_custom_shortcode($atts, $content = null) {
// Shortcode logic goes here
return '<div class="custom-content">' . $content . '</div>';
}
Register the shortcode
Use the add_shortcode() function to register your shortcode with WordPress. This typically goes in your theme’s functions.php file or a custom plugin:
add_shortcode('my_shortcode', 'my_custom_shortcode');
Use the shortcode in your content
Once registered, you can use your shortcode in posts and pages:
[my_shortcode]This is custom content[/my_shortcode]
Add attributes for flexibility
To make your shortcode more versatile, you can add attributes:
function my_custom_shortcode($atts, $content = null) {
$attributes = shortcode_atts(array(
'color' => 'blue',
'size' => 'medium'
), $atts);
return '<div class="custom-content" style="color:' . esc_attr($attributes['color']) . '; font-size:' . esc_attr($attributes['size']) . ';">' . $content . '</div>';
}
Now you can use attributes in your shortcode:
[my_shortcode color="red" size="large"]Custom styled content[/my_shortcode]
Enhance shortcodes with advanced features
As you become more comfortable with shortcode development, you can add more advanced features like:
- Nested shortcodes
- Database queries
- Integration with external APIs
- Dynamic content generation based on user roles or other conditions
The key is to identify repetitive tasks or complex features in your website and encapsulate them into easy-to-use shortcodes, making content creation more efficient and accessible for all users.
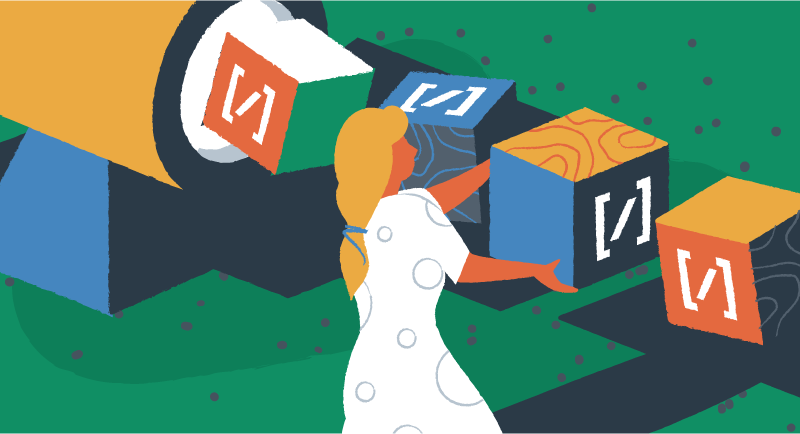
Best practices for WordPress shortcode development
When developing custom shortcodes for WordPress, following best practices is crucial to ensure your code is efficient, secure, and maintainable. Here are some key guidelines to keep in mind:
- Use unique prefixes: To avoid conflicts with other plugins or themes, prefix your shortcode names with something unique to your project:
add_shortcode('myproject_feature', 'myproject_feature_shortcode');
- Always return, never echo: Shortcodes should return their output, not echo it. This ensures proper integration with WordPress’s content flow:
function myproject_feature_shortcode($atts, $content = null) {
// Process shortcode
return $output; // Don't use echo here
}
Sanitize inputs and escape outputs: Always sanitize any user input and escape output to prevent security vulnerabilities:
$clean_input = sanitize_text_field($atts['user_input']);
$safe_output = esc_html($processed_content);
Use shortcode_atts() for default attributes: This function helps manage default values for your shortcode attributes:
$atts = shortcode_atts(array(
'color' => 'blue',
'size' => 'medium'
), $atts, 'myproject_feature');
Handle both self-closing and enclosing shortcodes: Make your shortcodes flexible by handling both formats:
function myproject_feature_shortcode($atts, $content = null) {
// Handle self-closing [myproject_feature]
if (is_null($content)) {
// Process without content
} else {
// Process with content
}
}
Optimize for performance: Avoid unnecessary database queries or heavy processing within shortcodes. If complex operations are needed, consider caching results:
$cache_key = 'myproject_feature_' . md5(serialize($atts));
$output = wp_cache_get($cache_key);
if (false === $output) {
// Generate output
wp_cache_set($cache_key, $output, 'myproject', 3600);
}
return $output;
Well-designed shortcodes should make life easier for both developers and end-users, so always strive for simplicity and clarity in your implementations.
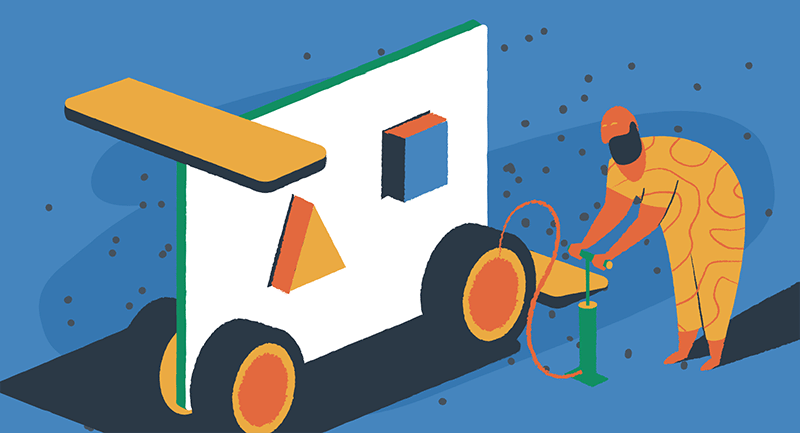
Troubleshooting common WordPress shortcode issues
You may encounter issues with WordPress shortcodes, even with careful development. Here are some common problems and their solutions to help you troubleshoot effectively:
- Shortcode not rendering: If your shortcode appears as plain text instead of rendering:
- Ensure the shortcode is properly registered with add_shortcode().
- Check for typos in the shortcode name.
- Verify that the shortcode function is returning, not echoing, content.
Solution:function my_shortcode($atts) {
return 'Shortcode content'; // Use return, not echo
}
add_shortcode('my_shortcode', 'my_shortcode');
- Conflicts with visual editors: Some visual editors may interfere with shortcode rendering:
- Use the text editor instead of the visual editor when inserting shortcodes.
- Wrap your shortcode in HTML comments to prevent editor interference:
<!-- [my_shortcode] -->
- Shortcode breaking page layout: If a shortcode disrupts your page layout:
- Check for unclosed HTML tags in your shortcode output.
- Ensure your shortcode doesn’t output content where it shouldn’t (e.g., in the <head> section).
Solution:function layout_safe_shortcode($atts) {
ob_start();
// Your shortcode content here
return ob_get_clean(); // Ensures clean output
}
add_shortcode('layout_safe', 'layout_safe_shortcode');
- Attributes not being recognized: If shortcode attributes aren’t working:
- Use shortcode_atts() to define default values and ensure proper attribute parsing.
Solution:function attribute_shortcode($atts) {
$args = shortcode_atts(array(
'color' => 'blue',
'size' => 'medium'
), $atts);
return "Color: {$args['color']}, Size: {$args['size']}";
}
add_shortcode('attribute_example', 'attribute_shortcode');
- Shortcode interfering with other plugins: If your shortcode conflicts with other plugins:
- Use unique prefixes for your shortcode names.
- Ensure your shortcode doesn’t output content prematurely.
Solution:function myproject_unique_shortcode($atts) {
// Your shortcode logic here
}
add_shortcode('myproject_feature', 'myproject_unique_shortcode');
- Shortcodes not working after theme switch: If shortcodes stop working after changing themes:
- Ensure your shortcodes are defined in a plugin, not the theme’s functions.php.
- If using theme-specific shortcodes, recreate them in the new theme.
Always test your shortcodes thoroughly in different contexts and with various content types to ensure they work as expected across your entire WordPress site.
Incorporate WordPress shortcodes with confidence
WordPress shortcodes are a powerful way to simplify complex functionality and easily enhance your site’s capabilities. Whether you use built-in shortcodes or create custom ones, these versatile tools can turn your website into a deeply customizable ecosystem.
As you explore the world of shortcodes, don’t stop here. Check out our complete guide to editing HTML, CSS, and PHP code in WordPress to take your site to the next level and create truly dynamic, personalized experiences for your visitors.